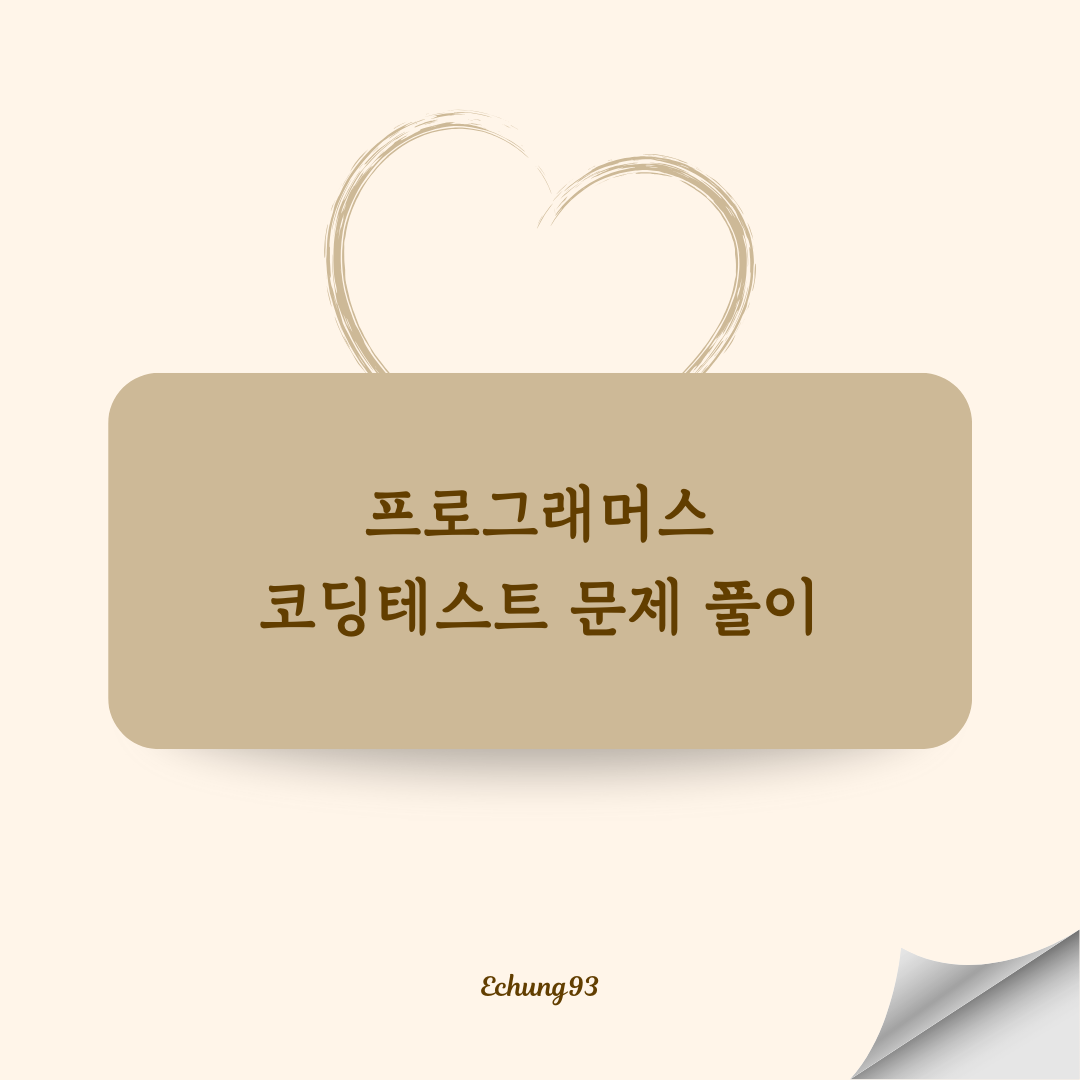
안녕하세요. 이번에는 프로그래머스 두 개 뽑아서 더하기 문제를 풀어보려고 합니다.
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
Problem
정수 배열 numbers가 주어집니다. numbers에서 서로 다른 인덱스에 있는 두 개의 수를 뽑아 더해서 만들 수 있는 모든 수를 배열에 오름차순으로 담아 return 하도록 solution 함수를 완성해 주세요.
[제한 사항]
○ numbers의 길이는 2 이상 100 이하입니다.
- numbers의 모든 수는 0 이상 100 이하입니다.
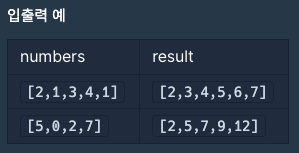
Solution
class Solution {
fun solution(numbers: IntArray): IntArray {
var hashSet = HashSet<Int>()
(numbers.indices).forEach {
i -> (i + 1 until numbers.size).forEach {
hashSet.add(numbers[i] + numbers[it])
}
}
return hashSet.sorted().toIntArray()
}
}
이번 문제는 hashSet을 사용해서 중복처리를 하는 것을 핵심으로 하여 문제를 풀어보았습니다.
1. 다른 사람 코드
class Solution {
fun solution(numbers: IntArray): IntArray {
val list = numbers.toList()
return list.withIndex().flatMap { i -> list.withIndex().map { j -> i to j } }
.filter { it.first.index != it.second.index }
.map { it.first.value + it.second.value }
.toSortedSet()
.toIntArray()
}
}
제가 문제를 접근한 방식이랑 비슷한 방식이지만 다른 함수를 사용해서 푼 문제를 가져와보았습니다. 여기서 withIndex()를 하는 코드는 list 값을 객체의 원소를 IndexedValue(index, value) 형태로 접근할 수 있도록 만들어주는 코드입니다.
코드 분석
list.withIndex().flatMap { i -> list.withIndex().map { j -> i to j } }
//위의 코드를 작동하면
//[(IndexedValue(index=0, value=2), IndexedValue(index=0, value=2)),
// (IndexedValue(index=0, value=2), IndexedValue(index=1, value=1)),
// ... (IndexedValue(Index=4, value=1), IndexedValue(index=4, value=1))
// 와 같은 코드로 나옵니다.
//이 코드를 서로 다른 인덱스를 선택하기 위해서 filter코드를 사용하였습니다.
.filter { it.first.index != it.second.index }
// filter 조건에 만족하는 값들을 Map을 사용해서 더해줍니다.
.map { it.first.value + it.second.value }
// sortedSet을 사용해서 정렬 및 중복 제거를 해줍니다.
.toSortedSet()
// 마지막으로 IntArray() 형태로 출력해줍니다.
.toIntArray()
코드에는 정답은 없지만 다른 사람의 코드를 분석하면서 새로운 함수들을 배우고 활용법을 배우는 것이 좋은 것 같습니다. 여러분들도 더 나은 코드가 있으면 언제든지 댓글로 남겨주시면 감사하겠습니다!
Performance
1. 내가 작성한 코드 | 2. 다른 사람 코드 |
![]() |
![]() |
반응형
'프로그래머스 Algorithm' 카테고리의 다른 글
[프로그래머스] 콜라 문제 Kotlin (2) | 2023.12.19 |
---|---|
[프로그래머스] 예상 대진표 Kotlin (2) | 2023.12.18 |
[프로그래머스] 푸드 파이트 대회 Kotlin (0) | 2023.12.16 |
[프로그래머스] 문자열 내 마음대로 정렬하기 Kotlin (2) | 2023.12.15 |
[프로그래머스] K번째 수 Kotlin (0) | 2023.12.14 |